No-code Screens
Leverage Qonversion's drag-and-drop mobile screen builder to build beautiful paywalls and onboarding flows. Increase user-to-trial, user-to-paid conversion rates, customer engagement and your app's revenue.
With Qonversion's remote no-code screens, you can:
- Build a single screen (e.g. paywall)
- Build a sequence of connected screens (e.g. onboarding)
- Track user actions like button clicks with your product analytics
- Edit screens' content remotely
1) Create a single screen
You can quickly create a beautiful paywall and other types of in-app screens with the Qonversion no-code screen builder. Follow this guide to learn the details of building the in-app screen similar to the examples below.
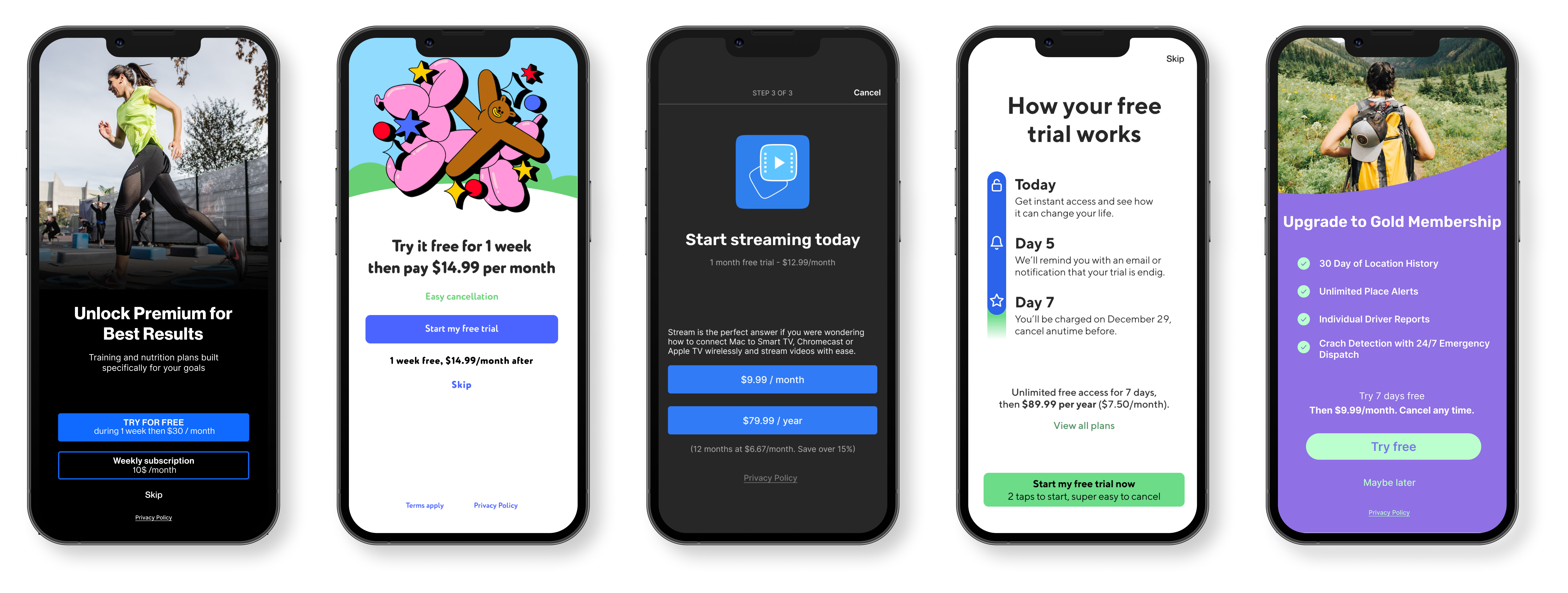
2. Create a sequence of screens
You can build a set of no-code screens using the guide above and link them into a sequence.
Simply connect your no-code screens using Navigate to the screen action in the screen builder.
Here is a short tutorial describing the sequence creation process:
3. Present a no-code screen
To present a no-code screen:
- Copy the screen's ID from the Qonversion dashboard
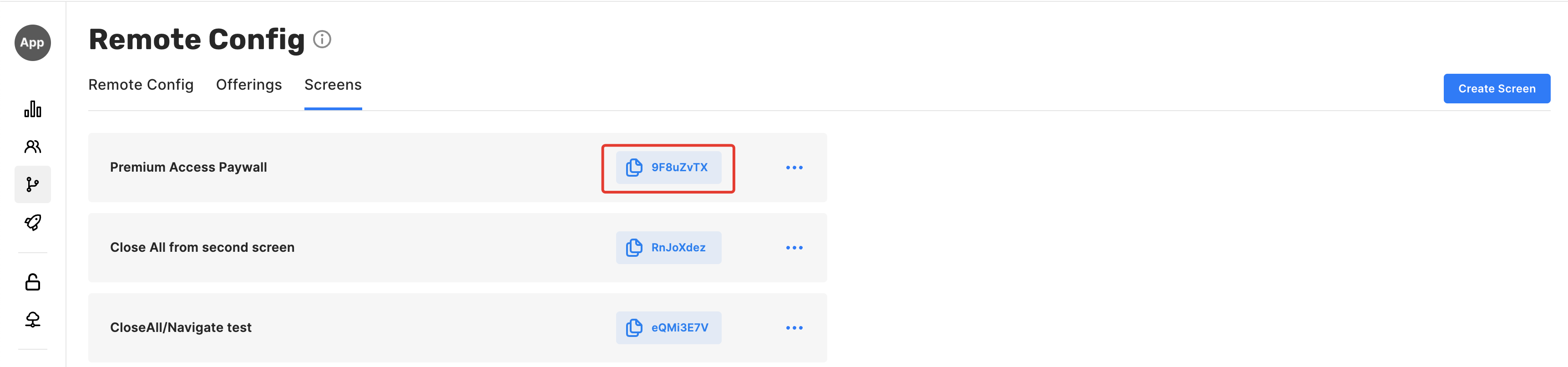
- Pass the ID to the following SDK method:
Qonversion.Automations.shared().showScreen(withID: "screen_id_from_the_dashboard") { success, error in
// handle success result or error here
}
[[QONAutomations sharedInstance] showScreenWithID:@"screen_id_from_the_dashboard" completion:^(BOOL success, NSError * _Nullable error) {
// handle success result or error here
}];
Automations.getSharedInstance().showScreen("screen_id_from_the_dashboard", new QonversionShowScreenCallback() {
@Override
public void onSuccess() {
// handle success here
}
@Override
public void onError(@NonNull QonversionError error) {
// handle error here
}
});
Automations.shared.showScreen("screen_id_from_the_dashboard", callback = object: QonversionShowScreenCallback {
override fun onSuccess() {
// handle success here
}
override fun onError(error: QonversionError) {
// handle error here
}
})
try {
await Automations.getSharedInstance().showScreen("screen_id_from_the_dashboard");
} catch (e) {
// handle error here
}
try {
await Automations.getSharedInstance().showScreen('screen_id_from_the_dashboard');
} catch (e) {
// handle error here
}
Automations.GetSharedInstance().ShowScreen("screen_id_from_the_dashboard", error =>
{
if (error != null)
{
// handle error here
}
});
try {
await Qonversion.Automations.getSharedInstance().showScreen('screen_id_from_the_dashboard');
} catch (e) {
// handle error here
}
- Call this method in any part of your app and enjoy remotely manageable no-code screens!
4) Customize screen presentation
You can configure with what animation a no-code screen should be presented. It works differently for native SDKs (iOS and Android) and for cross-platform SDKs. Let's have a look at them in turn.
Below are the minimal required SDK versions supporting this feature:
Platform | Minimal version |
---|---|
iOS | 3.1.0 |
Android | 4.1.0 |
React Native | 4.1.0 |
Flutter | 5.1.0 |
Unity | 4.1.0 |
For iOS and Android, you should provide a ScreenCustomizationDelegate
, which will be called each time before showing a no-code screen.
We use a weak reference to the provided delegate to protect against memory leaks, so ensure that your delegate will remain alive as long as you need it (keep the reference to it somewhere in your code).
// set the delegate somewhere in your code and keep the reference
Qonversion.Automations.shared().setScreenCustomizationDelegate(self)
...
// implement the function to return screen presentation configuration
func presentationConfigurationForScreen(_ screenId: String) -> Qonversion.ScreenPresentationConfiguration {
return Qonversion.ScreenPresentationConfiguration(presentationStyle: .popover, animated: true)
}
// set the delegate somewhere in your code and keep the reference
[[QONAutomations sharedInstance] setScreenCustomizationDelegate:self];
...
// implement the function to return screen presentation configuration
- (QONScreenPresentationConfiguration *)presentationConfigurationForScreen:(NSString *)screenId {
return [[QONScreenPresentationConfiguration alloc] initWithPresentationStyle:QONScreenPresentationStylePopover animated:YES];
}
// implement the delegate to return screen presentation configuration
// do not forget to keep the reference on this object
private final ScreenCustomizationDelegate screenCustomizationDelegate = new ScreenCustomizationDelegate() {
@NonNull
@Override
public QScreenPresentationConfig getPresentationConfigurationForScreen(@NonNull String screenId) {
if (screenId.equals("screen_id_from_the_dashboard")) {
// return configuration for the concrete screen
return new QScreenPresentationConfig(QScreenPresentationStyle.NoAnimation);
}
// return default configuration for all the rest screens
return new QScreenPresentationConfig(QScreenPresentationStyle.FullScreen);
}
};
...
// set the delegate somewhere in your code
Automations.getSharedInstance().setScreenCustomizationDelegate(screenCustomizationDelegate);
// implement the delegate to return screen presentation configuration
// do not forget to keep the reference on this object
private val screenCustomizationDelegate = object : ScreenCustomizationDelegate {
override fun getPresentationConfigurationForScreen(screenId: String): QScreenPresentationConfig {
if (screenId === "screen_id_from_the_dashboard") {
// return configuration for the concrete screen
return QScreenPresentationConfig(QScreenPresentationStyle.NoAnimation)
}
// return default configuration for all the rest screens
return QScreenPresentationConfig(QScreenPresentationStyle.FullScreen)
}
}
...
// set the delegate somewhere in your code
Automations.shared.setScreenCustomizationDelegate(screenCustomizationDelegate)
In cross-platform SDKs, for that purpose, you should call the setScreenPresentationConfig
method and provide a presentation configuration along with an identifier of a screen (optional) to which it should be applied. You can omit screen ID, and the provided configuration will be used for all no-code screens.
var config = new QScreenPresentationConfig(QScreenPresentationStyle.fullScreen, true);
// Set configuration for all screens.
Automations.getSharedInstance().setScreenPresentationConfig(config);
// Set configuration for the concrete screen.
Automations.getSharedInstance().setScreenPresentationConfig(config, "screen_id_from_the_dashboard");
const config = new ScreenPresentationConfig(ScreenPresentationStyle.FULL_SCREEN, true);
// Set configuration for all screens.
Automations.getSharedInstance().setScreenPresentationConfig(config);
// Set configuration for the concrete screen.
Automations.getSharedInstance().setScreenPresentationConfig(config, 'screen_id_from_the_dashboard');
ScreenPresentationConfig config = new ScreenPresentationConfig(ScreenPresentationStyle.FullScreen, true);
// Set configuration for all screens.
Automations.GetSharedInstance().SetScreenPresentationConfig(config);
// Set configuration for the concrete screen.
Automations.GetSharedInstance().SetScreenPresentationConfig(config, "screen_id_from_the_dashboard");
const config = new Qonversion.ScreenPresentationConfig(Qonversion.ScreenPresentationStyle.FULL_SCREEN, true);
// Set configuration for all screens.
Qonversion.Automations.getSharedInstance().setScreenPresentationConfig(config);
// Set configuration for the concrete screen.
Qonversion.Automations.getSharedInstance().setScreenPresentationConfig(config, 'screen_id_from_the_dashboard');
Call order mattersNote that the
setScreenPresentationConfig
call order matters. So if you call it for the concrete screen first and then for all the screens (without providing screen ID), the first call will be overridden.
The ScreenPresentationConfig
class consists of the following fields:
Field | Type | Description |
---|---|---|
|
| Describes how screens will be displayed. |
| boolean (default - | iOS only. A flag that enables/disables screen presentation animation.
For Android consider using |
ScreenPresentationStyle
is an enumeration of the following variants:
Variant | iOS description | Android description |
---|---|---|
Popover |
| Not available for Android |
Push | Not a modal representation. Pushes a controller to a current navigation stack. NavigationController on the top of the stack is required. | Default screen transaction animation on Android. |
FullScreen |
| A screen moves from bottom to top. Example. |
NoAnimation | Not available for iOS. For iOS consider providing | A screen appears/disappears without any animation. Example. |
Cross-platform attentionPlease pay attention that the
Push
screen presentation style for iOS requires the navigation controller in the current stack of your application views. To use thePush
presentation style, you must ensure your top-level view stack hasUINavigationController
. Otherwise, the no-code screen will not be presented.
5. Track no-code screen user clicks with analytics
In case you want to log button clicks or action results, add an Automations delegate to receive callbacks with the required information about users' behaviour.
Updated 8 days ago